Getting Started with Frames v2
Not ready to build?
If you'd prefer to learn more about the new spec before building a frame, jump ahead to the Specification.
Video demo
Here's a full walkthrough of creating a frames v2 app:
Tutorial
The following tutorial is based on the Frames v2 Demo repo on Github.
Setup and dependencies
We'll start with a fresh NextJS app:
$ yarn create next-app
✔ What is your project named? … frames-v2-demo
✔ Would you like to use TypeScript? … No / Yes
✔ Would you like to use ESLint? … No / Yes
✔ Would you like to use Tailwind CSS? … No / Yes
✔ Would you like your code inside a `src/` directory? … No / Yes
✔ Would you like to use App Router? (recommended) … No / Yes
✔ Would you like to use Turbopack for next dev? … No / Yes
✔ Would you like to customize the import alias (@/* by default)? … No / Yes
✔ What import alias would you like configured? … ~/*
Creating a new Next.js app in /Users/horsefacts/Projects/frames-v2-demo.
Next, install frame related dependencies. We'll need the official frame SDK:
$ yarn add @farcaster/frame-sdk
We'll also need Wagmi to handle wallet interactions. Let's install it and its dependencies.
$ yarn add wagmi viem@2.x @tanstack/react-query
OK, we're ready to get started!
Configuring providers
We'll need to set up a custom Wagmi connector in order to interact with the user's Farcaster wallet. Since the frames SDK is a frontend only package, we'll also need to use client components and Next dynamic imports in a few places.
First, let's create a custom connector component at lib/connector.ts
. We'll use this to connect to the user's Farcaster wallet from our app.
NOTE
We plan to move this connector into the frames SDK so you don't have to worry about it. But you'll need to copy-paste it for now.
import sdk from '@farcaster/frame-sdk';
import { SwitchChainError, fromHex, getAddress, numberToHex } from 'viem';
import { ChainNotConfiguredError, createConnector } from 'wagmi';
frameConnector.type = 'frameConnector' as const;
export function frameConnector() {
let connected = true;
return createConnector<typeof sdk.wallet.ethProvider>((config) => ({
id: 'farcaster',
name: 'Farcaster Wallet',
type: frameConnector.type,
async setup() {
this.connect({ chainId: config.chains[0].id });
},
async connect({ chainId } = {}) {
const provider = await this.getProvider();
const accounts = await provider.request({
method: 'eth_requestAccounts',
});
let currentChainId = await this.getChainId();
if (chainId && currentChainId !== chainId) {
const chain = await this.switchChain!({ chainId });
currentChainId = chain.id;
}
connected = true;
return {
accounts: accounts.map((x) => getAddress(x)),
chainId: currentChainId,
};
},
async disconnect() {
connected = false;
},
async getAccounts() {
if (!connected) throw new Error('Not connected');
const provider = await this.getProvider();
const accounts = await provider.request({
method: 'eth_requestAccounts',
});
return accounts.map((x) => getAddress(x));
},
async getChainId() {
const provider = await this.getProvider();
const hexChainId = await provider.request({ method: 'eth_chainId' });
return fromHex(hexChainId, 'number');
},
async isAuthorized() {
if (!connected) {
return false;
}
const accounts = await this.getAccounts();
return !!accounts.length;
},
async switchChain({ chainId }) {
const provider = await this.getProvider();
const chain = config.chains.find((x) => x.id === chainId);
if (!chain) throw new SwitchChainError(new ChainNotConfiguredError());
await provider.request({
method: 'wallet_switchEthereumChain',
params: [{ chainId: numberToHex(chainId) }],
});
return chain;
},
onAccountsChanged(accounts) {
if (accounts.length === 0) this.onDisconnect();
else
config.emitter.emit('change', {
accounts: accounts.map((x) => getAddress(x)),
});
},
onChainChanged(chain) {
const chainId = Number(chain);
config.emitter.emit('change', { chainId });
},
async onDisconnect() {
config.emitter.emit('disconnect');
connected = false;
},
async getProvider() {
return sdk.wallet.ethProvider;
},
}));
}
Next, let's create a provider component that handles our Wagmi configuration. Create components/providers/WagmiProvider.tsx
.
We'll configure our client with Base as a connected network and use the frameConnector
that we just created:
import { createConfig, http, WagmiProvider } from 'wagmi';
import { base } from 'wagmi/chains';
import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
import { frameConnector } from '~/lib/connector';
export const config = createConfig({
chains: [base],
transports: {
[base.id]: http(),
},
connectors: [frameConnector()],
});
const queryClient = new QueryClient();
export default function Provider({ children }: { children: React.ReactNode }) {
return (
<WagmiProvider config={config}>
<QueryClientProvider client={queryClient}>{children}</QueryClientProvider>
</WagmiProvider>
);
}
Now let's create a top-level Providers
component that will include all our required providers. In this simple demo app, we'll just be adding Wagmi, but this is where you might also add other providers necessary for your own app.
Create app/providers.tsx
:
'use client';
import dynamic from 'next/dynamic';
const WagmiProvider = dynamic(
() => import('~/components/providers/WagmiProvider'),
{
ssr: false,
}
);
export function Providers({ children }: { children: React.ReactNode }) {
return <WagmiProvider>{children}</WagmiProvider>;
}
Note two new things here: since the SDK relies on the browser window
, we need to define this as a client component with "use client";
and use a dynamic import to import WagmiProvider
.
Finally, let's add this providers component to our app layout. Edit app/layout.tsx
:
import type { Metadata } from 'next';
import '~/app/globals.css';
import { Providers } from '~/app/providers';
export const metadata: Metadata = {
title: 'Farcaster Frames v2 Demo',
description: 'A Farcaster Frames v2 demo app',
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body>
<Providers>{children}</Providers>
</body>
</html>
);
}
OK, setup is all done, let's do something more interesting...
Creating the app
Let's create a component for our app's homeUrl
page. Create app/components/Demo.tsx
.
For now, let's just put in a placeholder, Since our frame app will be rendering at mobile width, we'll give it a fixed width and center the content:
export default function Demo() {
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
</div>
);
}
Since we're going to import the frames SDK in this component, we'll need to load it dynamically, too. Edit app/page.tsx
:
'use client';
import dynamic from 'next/dynamic';
const Demo = dynamic(() => import('~/components/Demo'), {
ssr: false,
});
export default function Home() {
return (
<main className="min-h-screen flex flex-col p-4">
<Demo />
</main>
);
}
OK, we're all set up! Now is a good time to try out our frames app in the developer playground. To do so, we'll use ngrok to access our local dev server over the internet.
First, run the dev server:
$ yarn dev
Next, start ngrok:
$ ngrok http http://localhost:3000
Tunneling gotchas
Some tunneling tools, like the ngrok free tier, insert an click-through interstitial between your dev server and the tunnel endpoint. Use a paid ngrok account or a different tool, like Tailscale funnel.
Now open the Frame Playground on Warpcast mobile, by visiting https://warpcast.com/~/developers/frame-playground.
Enter your ngrok URL:
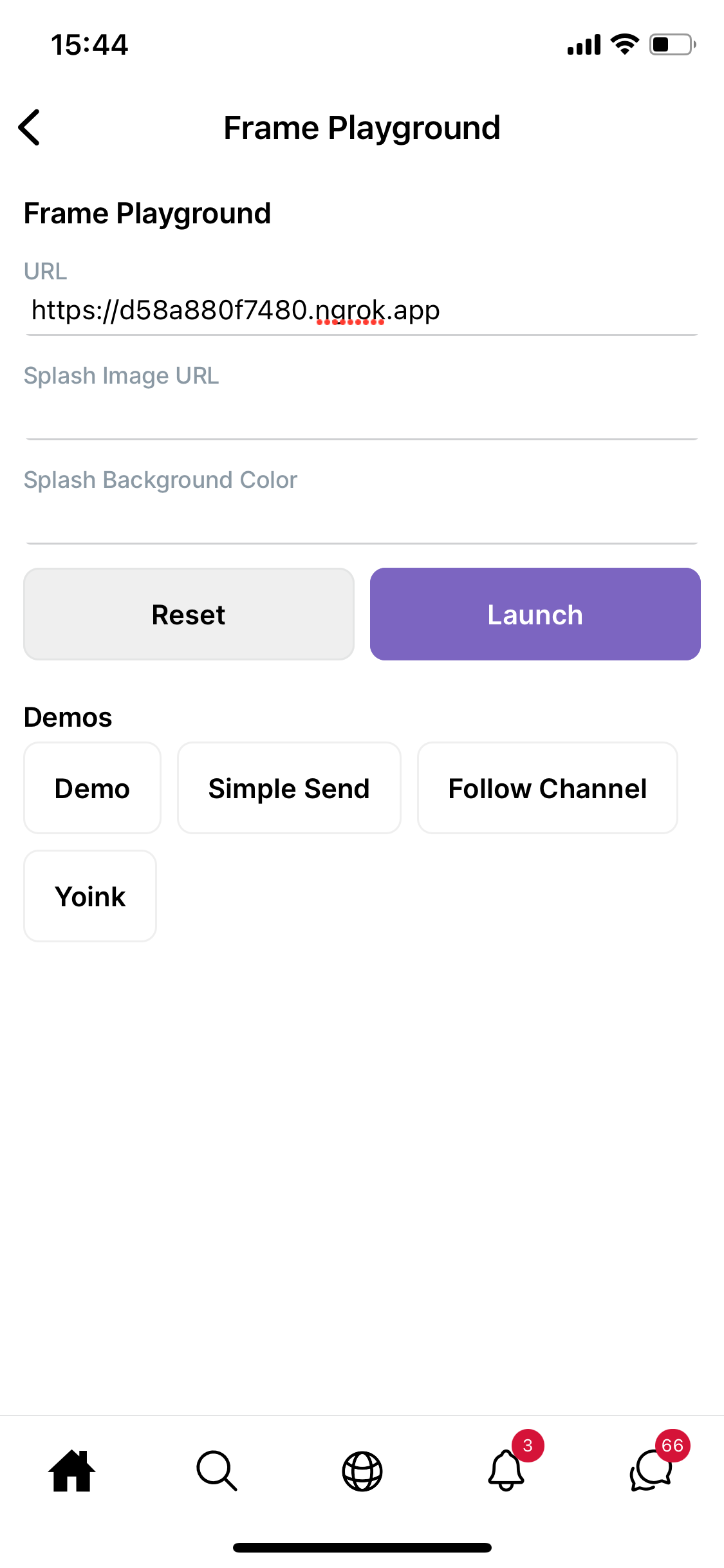
..and tap "Launch" to open your app.
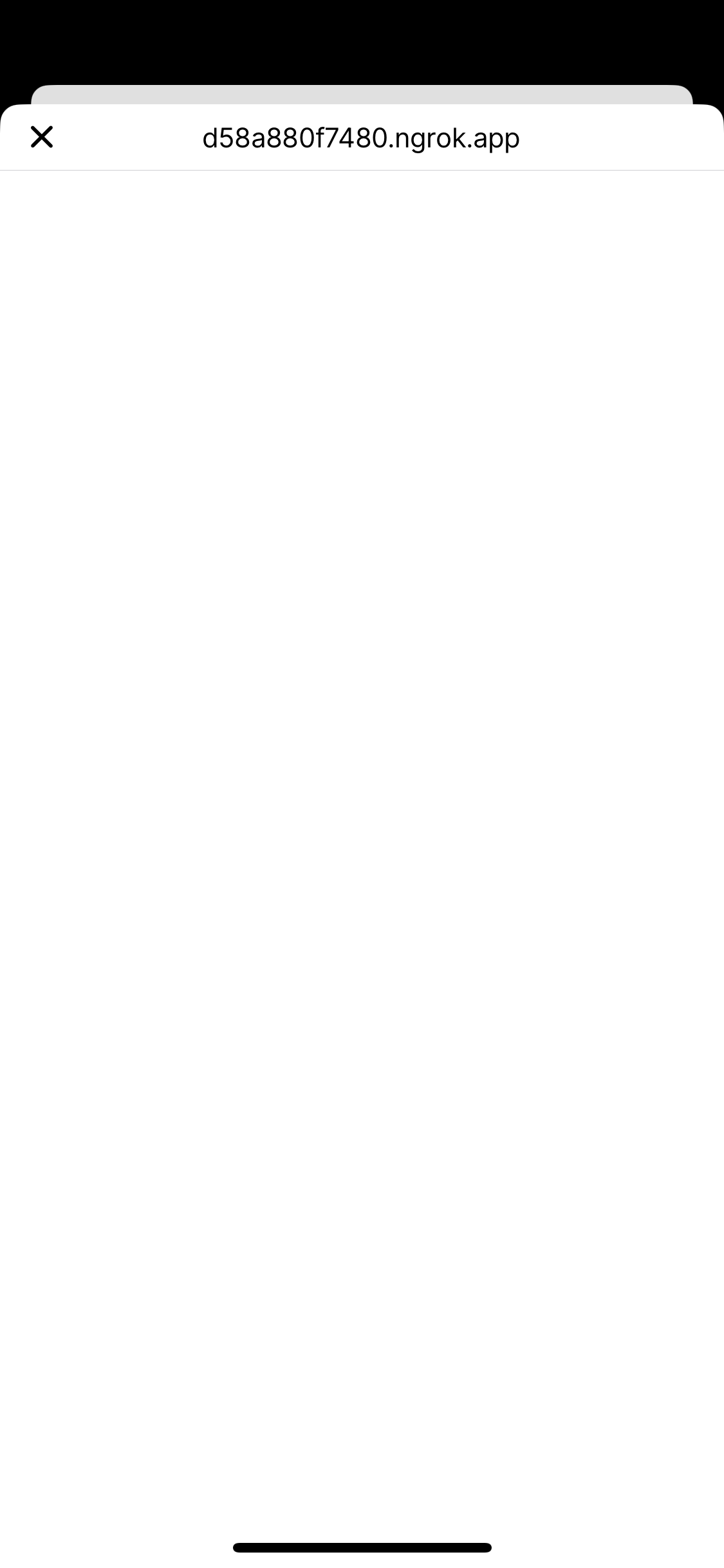
If you watch your dev server and ngrok logs, you'll see a request to your server. But nothing will load until we signal to Warpcast that our app is ready()
.
Calling ready()
To give frames a consistent loading experience, clients display a splash screen and image until the app calls sdk.actions.ready()
. In order to make it more visible here, let's add a splash image and loading color:
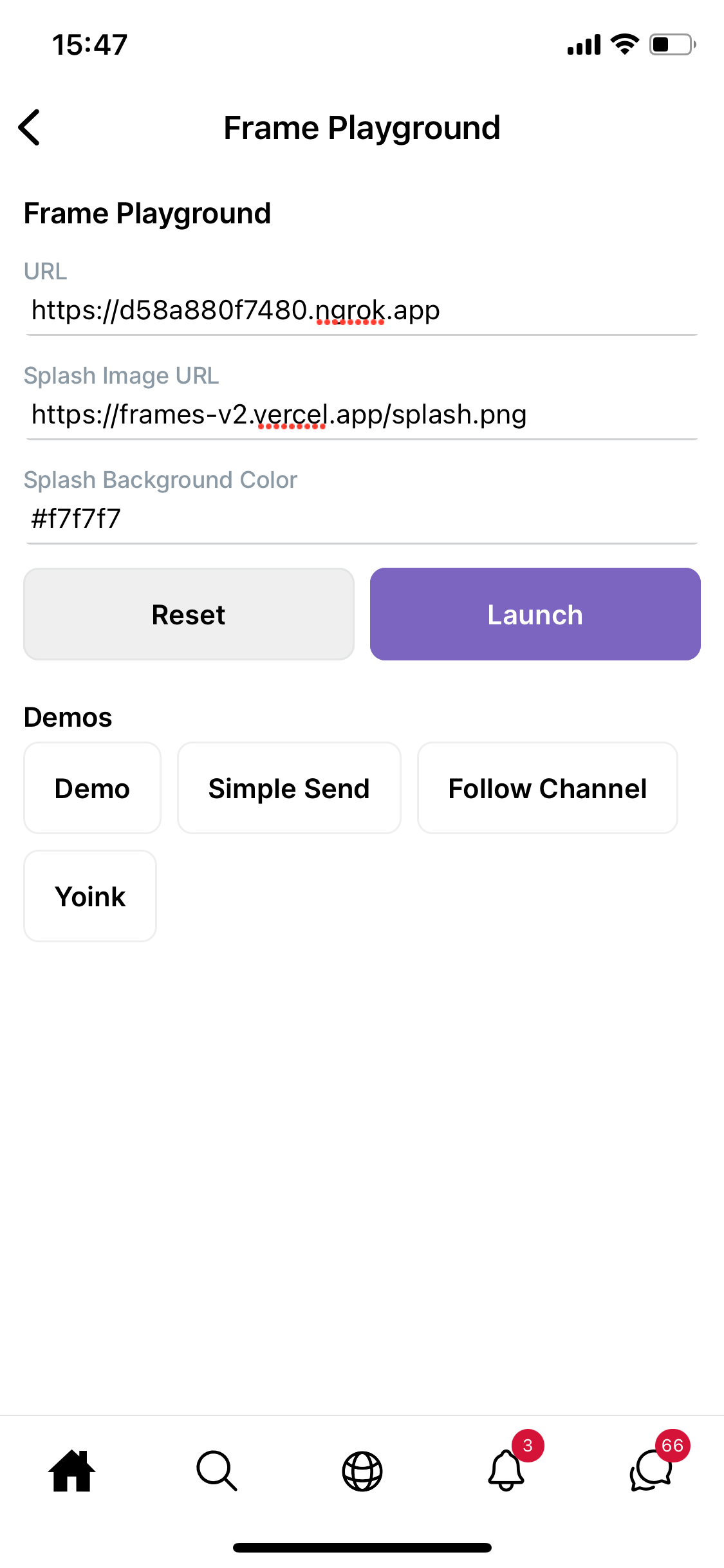
Now we get a nice background color and splash image:
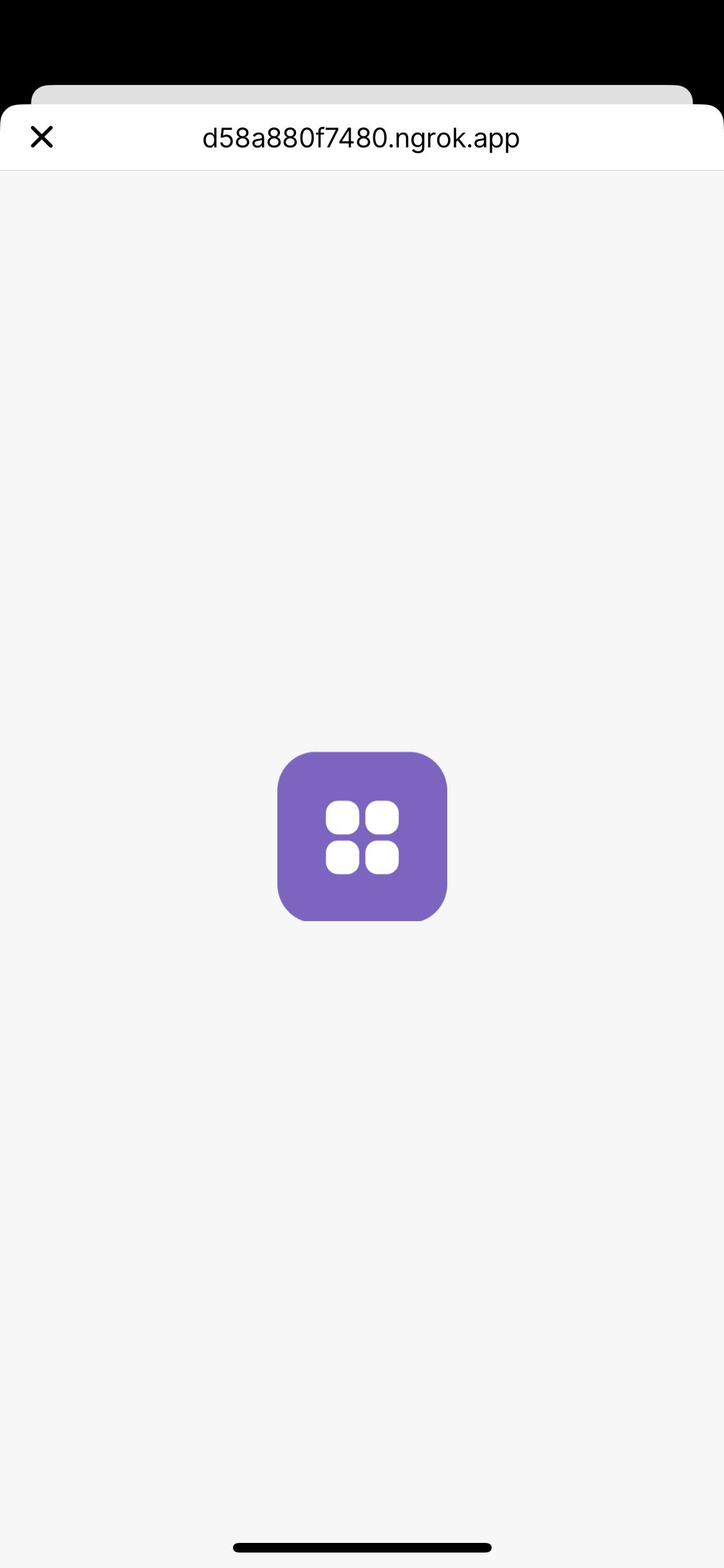
Let's call ready()
to load our app. We'll call sdk.actions.ready()
in an effect on render, which tells the parent Farcaster app that our frame is ready to render and hides the splash screen:
import { useEffect, useState } from 'react';
import sdk from '@farcaster/frame-sdk';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
useEffect(() => {
const load = async () => {
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
</div>
);
}
Try again in the playground and we'll see our app:
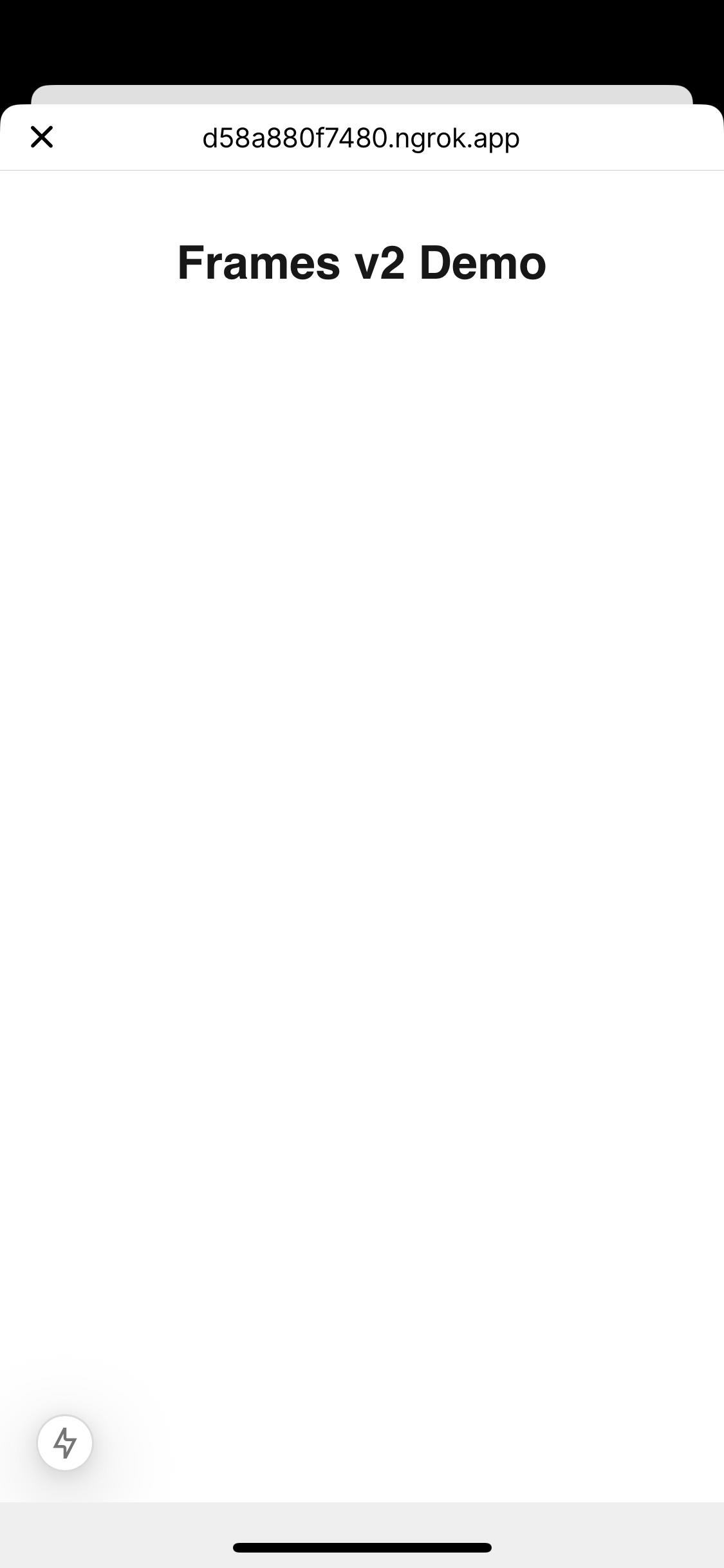
Viewing context
When your frame loads, the parent Farcaster app provides it with context information, including the current user. Let's take a look at it.
We can access the context data at sdk.context
to see information about the current user.:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
<div className="mb-4">
<h2 className="font-2xl font-bold">Context</h2>
<button
onClick={toggleContext}
className="flex items-center gap-2 transition-colors"
>
<span
className={`transform transition-transform ${
isContextOpen ? 'rotate-90' : ''
}`}
>
➤
</span>
Tap to expand
</button>
{isContextOpen && (
<div className="p-4 mt-2 bg-gray-100 dark:bg-gray-800 rounded-lg">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
{JSON.stringify(context, null, 2)}
</pre>
</div>
)}
</div>
</div>
);
}
When you load this in the Warpcast frames playground, you should see your own Farcaster user profile:
WARNING
In the current developer preview, context data is unauthenticated. Assume this data is spoofable and don't use it to grant privileged access to the user! Future frame SDK releases will include a mechanism fo verify context data.
This is a lot of data, so let's hide it behind a simple toggle:
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const [isContextOpen, setIsContextOpen] = useState(false);
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
const toggleContext = useCallback(() => {
setIsContextOpen((prev) => !prev);
}, []);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
<div className="mb-4">
<h2 className="font-2xl font-bold">Context</h2>
<button
onClick={toggleContext}
className="flex items-center gap-2 transition-colors"
>
<span
className={`transform transition-transform ${
isContextOpen ? 'rotate-90' : ''
}`}
>
➤
</span>
Tap to expand
</button>
{isContextOpen && (
<div className="p-4 mt-2 bg-gray-100 dark:bg-gray-800 rounded-lg">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
{JSON.stringify(context, null, 2)}
</pre>
</div>
)}
</div>
</div>
);
}
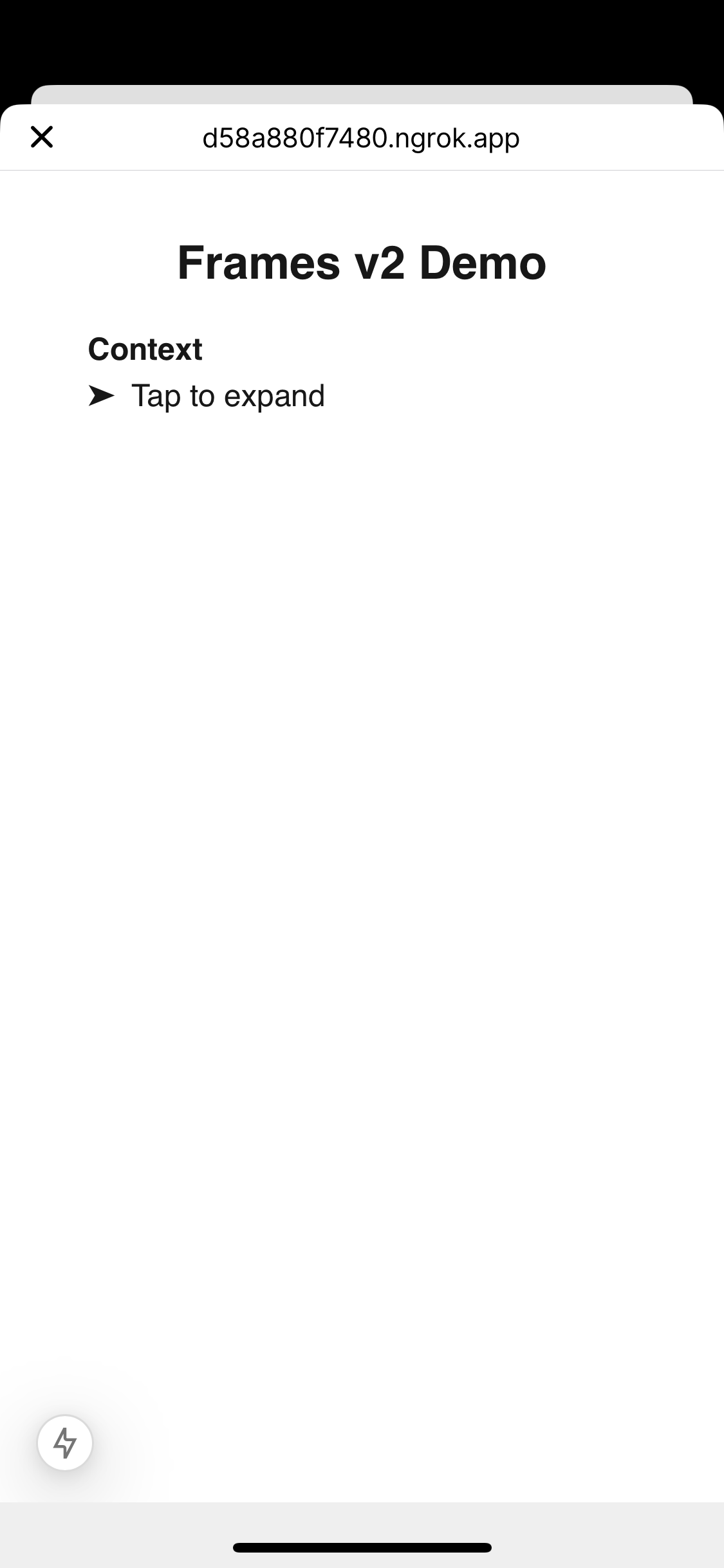
Invoking actions
Now let's make our frame do something. We can invoke actions by calling the functions on sdk.actions
. We've already used sdk.actions.ready
. We can also call functions like sdk.actions.openUrl
and sdk.actions.close
to send commands back to the Farcaster client app.
Let's start by opening an external URL. Add an openUrl
callback that calls sdk.actions.openUrl
and a button that calls it:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const [isContextOpen, setIsContextOpen] = useState(false);
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
const openUrl = useCallback(() => {
sdk.actions.openUrl('https://www.youtube.com/watch?v=dQw4w9WgXcQ');
}, []);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
{/* context toggle and data */}
<div>
<h2 className="font-2xl font-bold">Actions</h2>
<div className="mb-4">
<div className="p-2 bg-gray-100 dark:bg-gray-800 rounded-lg my-2">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
sdk.actions.openUrl
</pre>
</div>
<Button onClick={openUrl}>Open Link</Button>
</div>
</div>
</div>
);
}
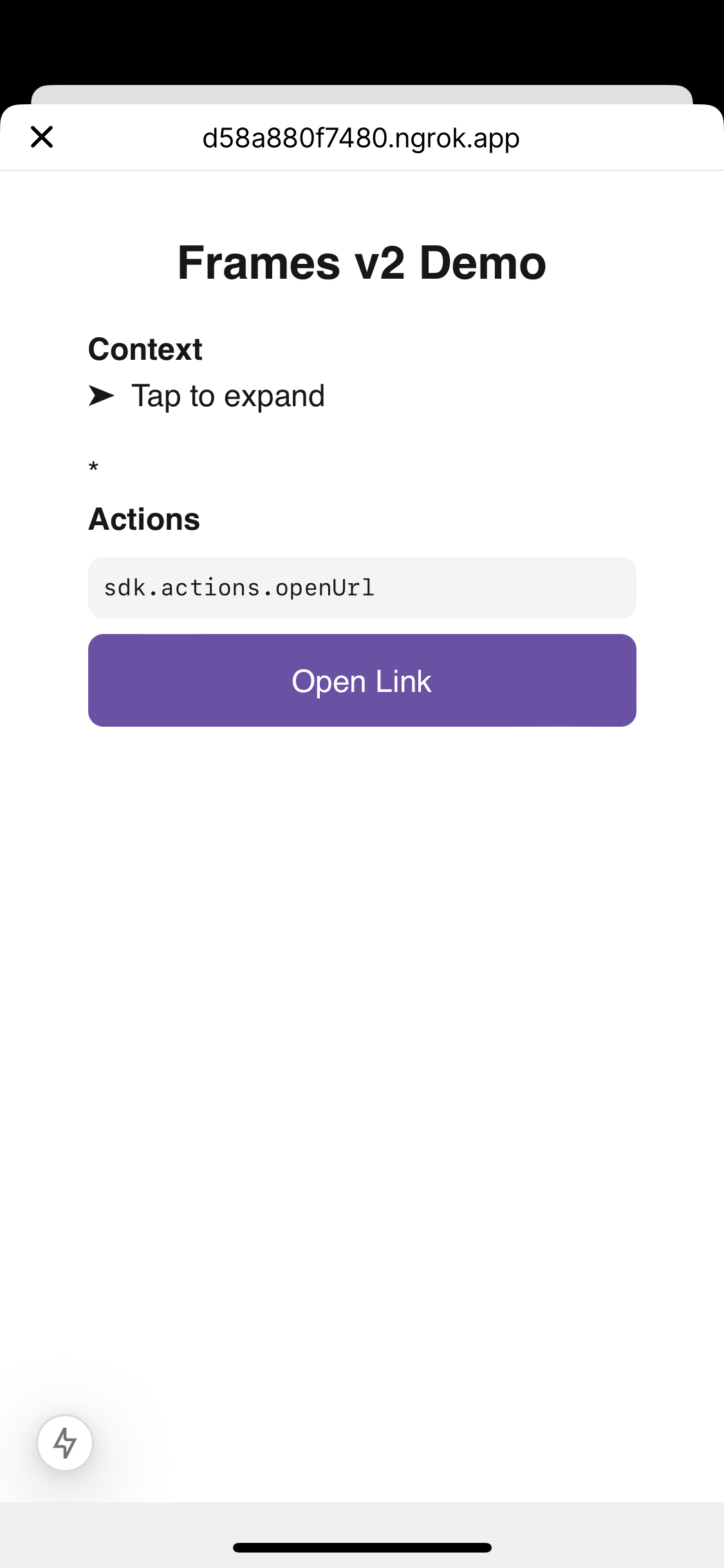
Tap the button and you'll be directed to an external URL.
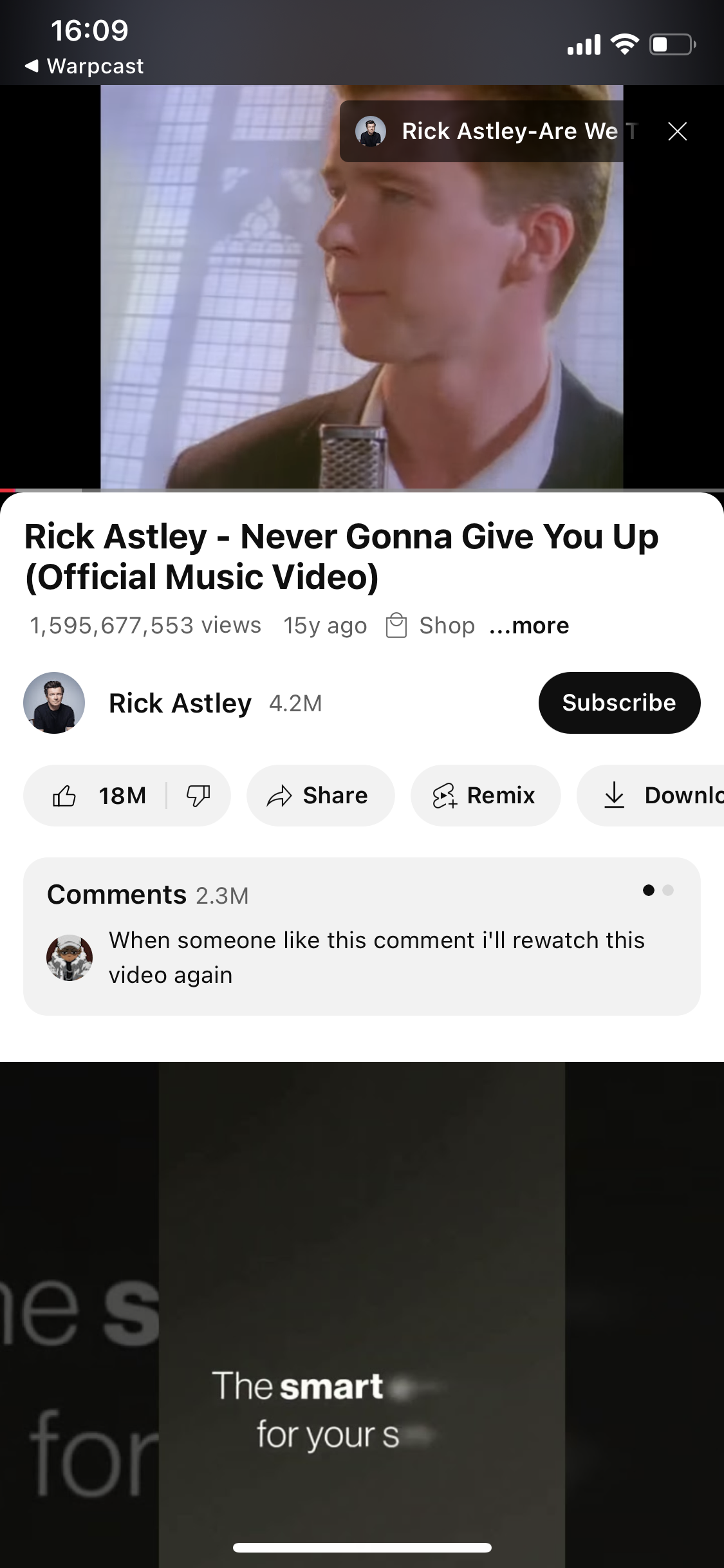
Let's add another button to call close()
:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
const openUrl = useCallback(() => {
sdk.actions.openUrl('https://www.youtube.com/watch?v=dQw4w9WgXcQ');
}, []);
const close = useCallback(() => {
sdk.actions.close();
}, []);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
<div>
<h2 className="font-2xl font-bold">Actions</h2>
<div className="mb-4">
<div className="p-2 bg-gray-100 dark:bg-gray-800 rounded-lg my-2">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
sdk.actions.openUrl
</pre>
</div>
<Button onClick={openUrl}>Open Link</Button>
</div>
<div className="mb-4">
<div className="p-2 bg-gray-100 dark:bg-gray-800 rounded-lg my-2">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
sdk.actions.close
</pre>
</div>
<Button onClick={close}>Close Frame</Button>
</div>
</div>
</div>
);
}
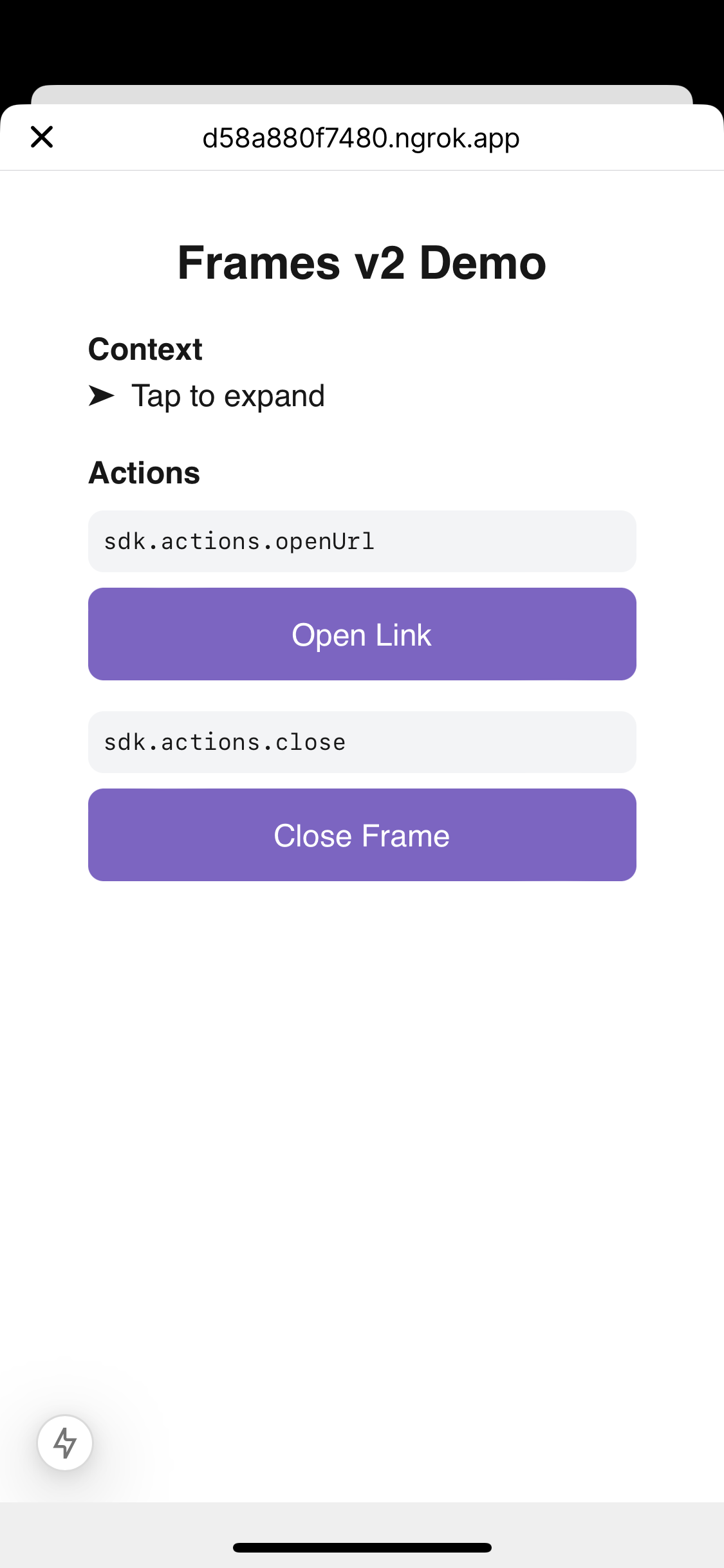
When you tap this, the frame should close.
Wallet interactions
Finally, let's interact with the user's connected wallet. To do so, we can use the wallet connector and Wagmi hooks we set up earlier. To start, let's read the user's connected wallet address, using useAccount
:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
import { useAccount } from 'wagmi';
import { Button } from '~/components/ui/Button';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const { address, isConnected } = useAccount();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
{/* Context and action buttons omitted */}
<div>
<h2 className="font-2xl font-bold">Wallet</h2>
{address && (
<div className="my-2 text-xs">
Address: <pre className="inline">{address}</pre>
</div>
)}
</div>
</div>
);
}

If your wallet is connected to Warpcast, you should see its address. In case it's not, let's add a connect/disconnect button. Note that we'll need to import our Wagmi config to connect
:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
import { useAccount } from 'wagmi';
import { config } from '~/components/providers/WagmiProvider';
import { Button } from '~/components/ui/Button';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const { address, isConnected } = useAccount();
const { disconnect } = useDisconnect();
const { connect } = useConnect();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
{/* Context and action buttons omitted */}
<div>
<h2 className="font-2xl font-bold">Wallet</h2>
{address && (
<div className="my-2 text-xs">
Address: <pre className="inline">{address}</pre>
</div>
)}
<div className="mb-4">
<Button
onClick={() =>
isConnected
? disconnect()
: connect({ connector: config.connectors[0] })
}
>
{isConnected ? 'Disconnect' : 'Connect'}
</Button>
</div>
</div>
</div>
);
}
Now let's request a transaction. We'll use the Wagmi useSendTransaction
hook to call the Yoink contract and useWaitForTransactionReceipt
to watch its status.
NOTE
In a more complex app, you'll probably want to use Wagmi's useWriteContract hook instead. This provides better type safety and automatic encoding/decoding of calldata based on the contract ABI.
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
import {
useAccount,
useSendTransaction,
useSignMessage,
useSignTypedData,
useWaitForTransactionReceipt,
useDisconnect,
useConnect,
} from 'wagmi';
import { config } from '~/components/providers/WagmiProvider';
import { Button } from '~/components/ui/Button';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const [txHash, setTxHash] = useState<string | null>(null);
const { address, isConnected } = useAccount();
const {
sendTransaction,
error: sendTxError,
isError: isSendTxError,
isPending: isSendTxPending,
} = useSendTransaction();
const { isLoading: isConfirming, isSuccess: isConfirmed } =
useWaitForTransactionReceipt({
hash: txHash as `0x${string}`,
});
const { disconnect } = useDisconnect();
const { connect } = useConnect();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
const sendTx = useCallback(() => {
sendTransaction(
{
to: '0x4bBFD120d9f352A0BEd7a014bd67913a2007a878',
data: '0x9846cd9efc000023c0',
},
{
onSuccess: (hash) => {
setTxHash(hash);
},
}
);
}, [sendTransaction]);
const renderError = (error: Error | null) => {
if (!error) return null;
return <div className="text-red-500 text-xs mt-1">{error.message}</div>;
};
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
{/* Context and actions omitted. */}
<div>
<h2 className="font-2xl font-bold">Wallet</h2>
{address && (
<div className="my-2 text-xs">
Address: <pre className="inline">{address}</pre>
</div>
)}
<div className="mb-4">
<Button
onClick={() =>
isConnected
? disconnect()
: connect({ connector: config.connectors[0] })
}
>
{isConnected ? 'Disconnect' : 'Connect'}
</Button>
</div>
{isConnected && (
<>
<div className="mb-4">
<Button
onClick={sendTx}
disabled={!isConnected || isSendTxPending}
isLoading={isSendTxPending}
>
Send Transaction
</Button>
{isSendTxError && renderError(sendTxError)}
{txHash && (
<div className="mt-2 text-xs">
<div>Hash: {txHash}</div>
<div>
Status:{' '}
{isConfirming
? 'Confirming...'
: isConfirmed
? 'Confirmed!'
: 'Pending'}
</div>
</div>
)}
</div>
</>
)}
</div>
</div>
);
}
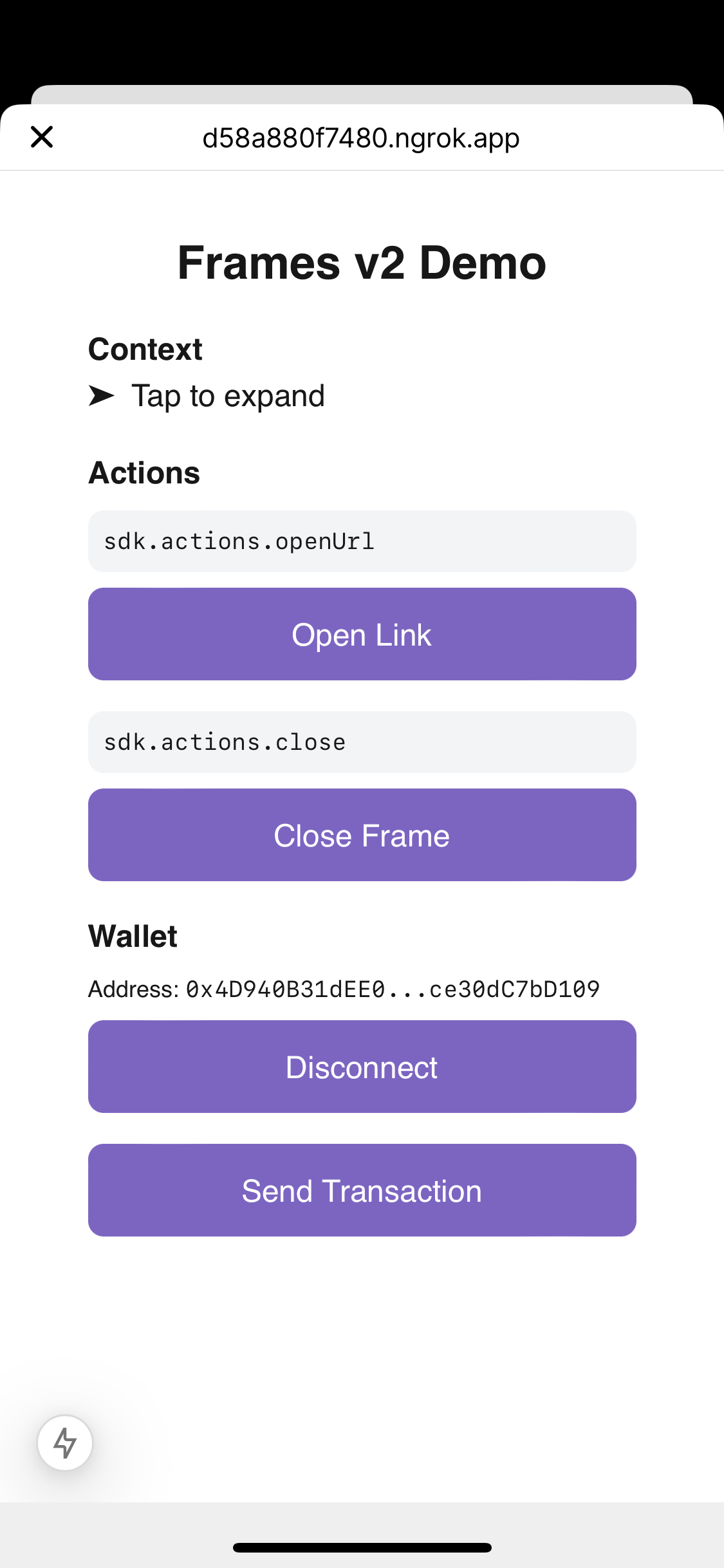
Tap "Send Transaction" and you'll be directed to your wallet.
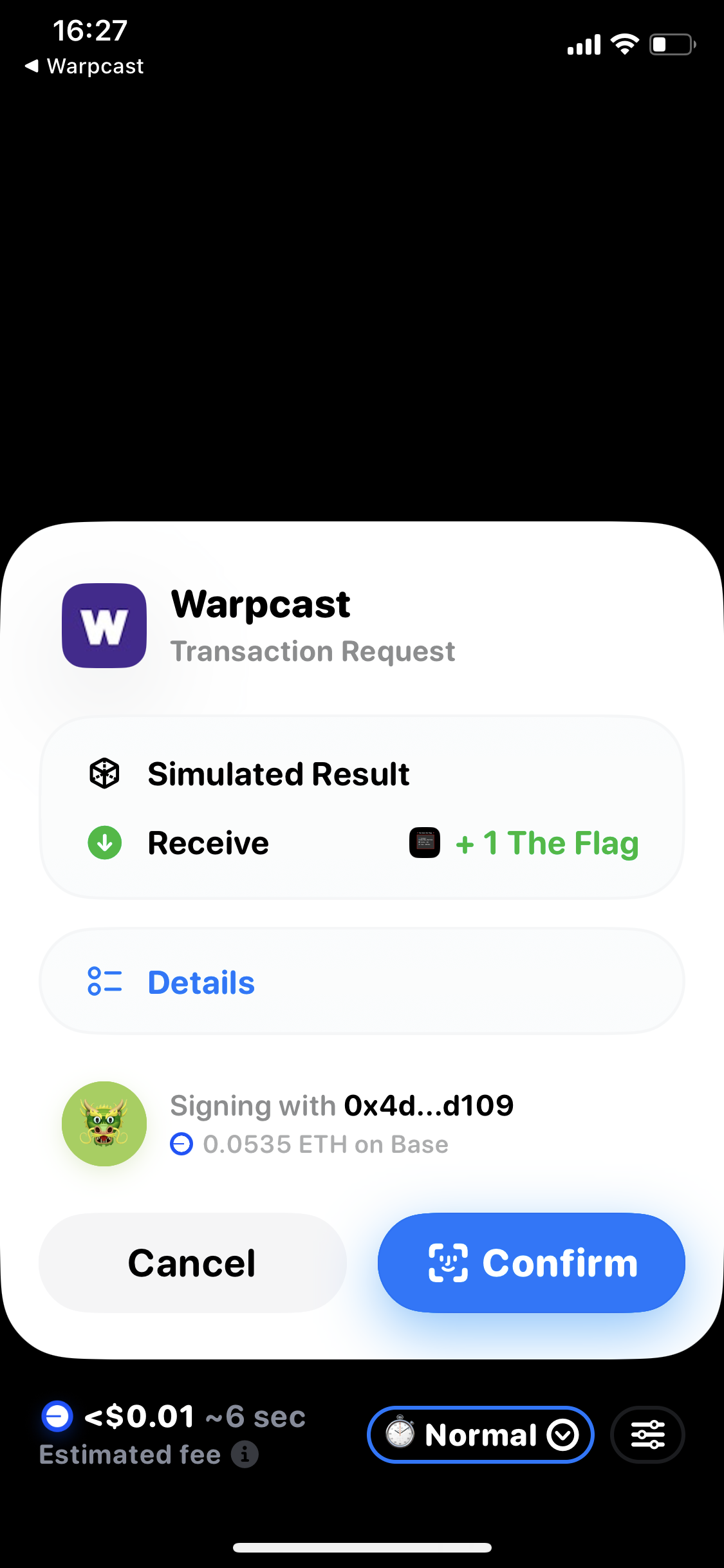
Signatures
Finally, let's add two new helpers for wallet signature methods. Below is the full Demo
component:
import { useEffect, useCallback, useState } from 'react';
import sdk, { type FrameContext } from '@farcaster/frame-sdk';
import {
useAccount,
useSendTransaction,
useSignMessage,
useSignTypedData,
useWaitForTransactionReceipt,
useDisconnect,
useConnect,
} from 'wagmi';
import { config } from '~/components/providers/WagmiProvider';
import { Button } from '~/components/ui/Button';
import { truncateAddress } from '~/lib/truncateAddress';
export default function Demo() {
const [isSDKLoaded, setIsSDKLoaded] = useState(false);
const [context, setContext] = useState<FrameContext>();
const [isContextOpen, setIsContextOpen] = useState(false);
const [txHash, setTxHash] = useState<string | null>(null);
const { address, isConnected } = useAccount();
const {
sendTransaction,
error: sendTxError,
isError: isSendTxError,
isPending: isSendTxPending,
} = useSendTransaction();
const { isLoading: isConfirming, isSuccess: isConfirmed } =
useWaitForTransactionReceipt({
hash: txHash as `0x${string}`,
});
const {
signMessage,
error: signError,
isError: isSignError,
isPending: isSignPending,
} = useSignMessage();
const {
signTypedData,
error: signTypedError,
isError: isSignTypedError,
isPending: isSignTypedPending,
} = useSignTypedData();
const { disconnect } = useDisconnect();
const { connect } = useConnect();
useEffect(() => {
const load = async () => {
setContext(await sdk.context);
sdk.actions.ready();
};
if (sdk && !isSDKLoaded) {
setIsSDKLoaded(true);
load();
}
}, [isSDKLoaded]);
const openUrl = useCallback(() => {
sdk.actions.openUrl('https://www.youtube.com/watch?v=dQw4w9WgXcQ');
}, []);
const close = useCallback(() => {
sdk.actions.close();
}, []);
const sendTx = useCallback(() => {
sendTransaction(
{
to: '0x4bBFD120d9f352A0BEd7a014bd67913a2007a878',
data: '0x9846cd9efc000023c0',
},
{
onSuccess: (hash) => {
setTxHash(hash);
},
}
);
}, [sendTransaction]);
const sign = useCallback(() => {
signMessage({ message: 'Hello from Frames v2!' });
}, [signMessage]);
const signTyped = useCallback(() => {
signTypedData({
domain: {
name: 'Frames v2 Demo',
version: '1',
chainId: 8453,
},
types: {
Message: [{ name: 'content', type: 'string' }],
},
message: {
content: 'Hello from Frames v2!',
},
primaryType: 'Message',
});
}, [signTypedData]);
const toggleContext = useCallback(() => {
setIsContextOpen((prev) => !prev);
}, []);
const renderError = (error: Error | null) => {
if (!error) return null;
return <div className="text-red-500 text-xs mt-1">{error.message}</div>;
};
if (!isSDKLoaded) {
return <div>Loading...</div>;
}
return (
<div className="w-[300px] mx-auto py-4 px-2">
<h1 className="text-2xl font-bold text-center mb-4">Frames v2 Demo</h1>
<div className="mb-4">
<h2 className="font-2xl font-bold">Context</h2>
<button
onClick={toggleContext}
className="flex items-center gap-2 transition-colors"
>
<span
className={`transform transition-transform ${
isContextOpen ? 'rotate-90' : ''
}`}
>
➤
</span>
Tap to expand
</button>
{isContextOpen && (
<div className="p-4 mt-2 bg-gray-100 dark:bg-gray-800 rounded-lg">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
{JSON.stringify(context, null, 2)}
</pre>
</div>
)}
</div>
<div>
<h2 className="font-2xl font-bold">Actions</h2>
<div className="mb-4">
<div className="p-2 bg-gray-100 dark:bg-gray-800 rounded-lg my-2">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
sdk.actions.openUrl
</pre>
</div>
<Button onClick={openUrl}>Open Link</Button>
</div>
<div className="mb-4">
<div className="p-2 bg-gray-100 dark:bg-gray-800 rounded-lg my-2">
<pre className="font-mono text-xs whitespace-pre-wrap break-words max-w-[260px] overflow-x-">
sdk.actions.close
</pre>
</div>
<Button onClick={close}>Close Frame</Button>
</div>
</div>
<div>
<h2 className="font-2xl font-bold">Wallet</h2>
{address && (
<div className="my-2 text-xs">
Address: <pre className="inline">{truncateAddress(address)}</pre>
</div>
)}
<div className="mb-4">
<Button
onClick={() =>
isConnected
? disconnect()
: connect({ connector: config.connectors[0] })
}
>
{isConnected ? 'Disconnect' : 'Connect'}
</Button>
</div>
{isConnected && (
<>
<div className="mb-4">
<Button
onClick={sendTx}
disabled={!isConnected || isSendTxPending}
isLoading={isSendTxPending}
>
Send Transaction
</Button>
{isSendTxError && renderError(sendTxError)}
{txHash && (
<div className="mt-2 text-xs">
<div>Hash: {truncateAddress(txHash)}</div>
<div>
Status:{' '}
{isConfirming
? 'Confirming...'
: isConfirmed
? 'Confirmed!'
: 'Pending'}
</div>
</div>
)}
</div>
<div className="mb-4">
<Button
onClick={sign}
disabled={!isConnected || isSignPending}
isLoading={isSignPending}
>
Sign Message
</Button>
{isSignError && renderError(signError)}
</div>
<div className="mb-4">
<Button
onClick={signTyped}
disabled={!isConnected || isSignTypedPending}
isLoading={isSignTypedPending}
>
Sign Typed Data
</Button>
{isSignTypedError && renderError(signTypedError)}
</div>
</>
)}
</div>
</div>
);
}
We've build a simple v2 frame by:
- Setting up a NextJS web app
- Importing the Frames SDK and calling
sdk.actions.ready()
- Reading the user context from
sdk.context
- Invoking actions using
sdk.actions
- Connecting to the user's wallet using Wagmi and
sdk.wallet.ethProvider